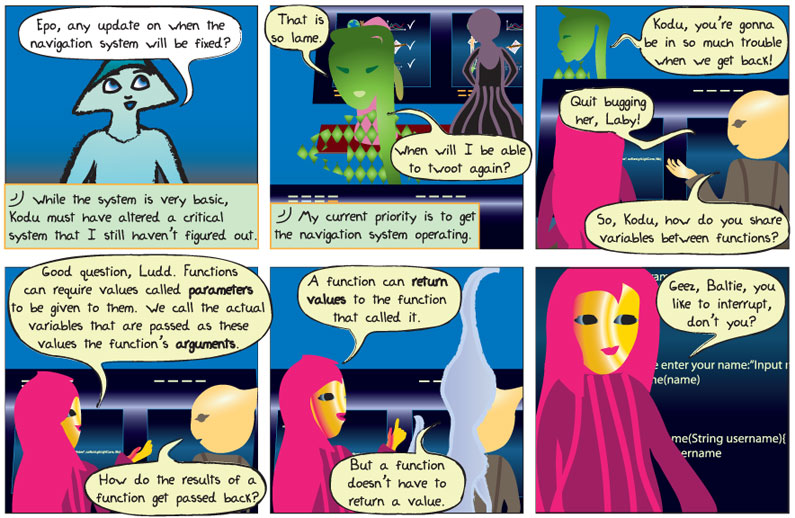
Comic Transcript
Panel 1.
Alkina: Epo, any update on when the navigation system will be fixed?
Epo: While the system is very basic, Kodu must have altered a critical system that I still haven’t figured out.
Panel 2.
Laby: That is so lame. When will I be able to twoot again?
Epo: My current priority is to get the navigation system operating.
Panel 3.
Laby: Kodu, you’re gonna be in so much trouble when we get back!
Ludd: Quit bugging her, Laby! So, Kodu, how do you share variables between functions?
Panel 4.
Kodu: Good question, Ludd. Functions can require values called parameters to be given to them. We call the actual variables that are passed as these values the function’s arguments.
Ludd: How do the results of a function get passed back?
Panel 5.
Kodu: A function can return values to the function that called it.
Baltie: But a function doesn’t have to return a value.
Panel 6.
Kodu: Geez, Baltie, you like to interrupt, don’t you?
Hide Transcript
What does it mean?
Parameter – a special type of variable that may be required as the input for a function. If the variable being passed to the function does not match the data type that the function expects, the program will produce an error.
Argument – is the variable that is being passed to a function.
Return value – this is the final result of a function that is returned to the function that initiated it.
In human speak please!
Now that we have discussed several of the basic building blocks of a computer program, let’s look at an example.
String name
Main {
Print “Please enter your name:”
Input name
DisplayName(name)
}
Void DisplayName(String username) {
Print “Hello “ + username
}
End Program
The program above does not use any particular programming language but should give you an idea of what a computer program looks like. Here is what each of the lines mean.
This is a declaration of a variable of the data type string, which is a string of characters.
A computer program can be made of several functions and they don’t necessarily have to be in the order in which they will run so the computer program needs to know where to start. In modern programming languages there is usually a “main” function, which is where the program starts running. The beginning and end of a function is usually indicated by curly braces “{“ and “},” respectively.
Just as the line indicates, this line displays the text “Please enter your name:” to the user. The word “Print” that starts the line would be a built-in function (one that programmers do not have to define themselves) of the programming language. It displays text to the user.
“Input,” in this case, is another built-in function. It takes input from the user and assigns it to the variable “name.”
This line calls the function “DisplayName” and passes it the argument “name” for the function to use.
This is the start of a new function. The word “Void” simply means that this function does not return a value. “String username” is the parameter that this function accepts, which, in this case is of the data type “String.” It is similar to declaring a variable inside a function definition. The reason that the variable “username” is used is because a function can have more than one parameter of data type “String” so each parameter has to be distinguished with its own variable. Variables can be pretty much anything. For example, instead of “username” we could have used “x.” However, it makes sense to use variables that make it easier for the programmer to know what each variable is. Similarly, it is a good habit to name functions by what they do. It is pretty easy to tell what the function “DisplayName” does simply from its name.
Can you figure out what this line of code does?
This is, of course, a very simple example that uses some of the ideas we have already talked about. In some ways, this program is more complicated than it needs to be, but this was done to illustrate the concepts we have talked about in recent episodes. How could you simplify this program?